Public Class frmMain
Private amount As Double
Private total As Double
Private Sub btnCalculate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCalculate.Click, btnCalculateChange.Click
Dim prices() As Double = {5, 10, 7, 15}
If lstItems.SelectedIndex <> -1 Then
Dim number As Integer
Integer.TryParse(txtNumber.Text, number)
Double.TryParse(txtAmount.Text, amount)
Dim subTotal As Double = number * prices(lstItems.SelectedIndex)
Dim VAT As Double = subTotal * 0.06
Dim discount As Double = subTotal * 0.1
total = subTotal + VAT - discount
txtVAT.Text = VAT.ToString("N2")
txtDiscount.Text = discount.ToString("N2")
txtTotal.Text = total.ToString("N2")
btnCalculateChange.Enabled = True
End If
End Sub
Private Sub btnCalculateChange_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCalculateChange.Click
Dim change As Double = amount - total
txtChange.Text = change.ToString("N2")
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class frmMain
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
Try
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
Finally
MyBase.Dispose(disposing)
End Try
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.lstItems = New System.Windows.Forms.ListBox()
Me.btnCalculate = New System.Windows.Forms.Button()
Me.Label1 = New System.Windows.Forms.Label()
Me.txtNumber = New System.Windows.Forms.TextBox()
Me.Label2 = New System.Windows.Forms.Label()
Me.txtVAT = New System.Windows.Forms.TextBox()
Me.Label3 = New System.Windows.Forms.Label()
Me.txtDiscount = New System.Windows.Forms.TextBox()
Me.Label4 = New System.Windows.Forms.Label()
Me.txtAmount = New System.Windows.Forms.TextBox()
Me.Label5 = New System.Windows.Forms.Label()
Me.txtTotal = New System.Windows.Forms.TextBox()
Me.Label6 = New System.Windows.Forms.Label()
Me.txtChange = New System.Windows.Forms.TextBox()
Me.btnCalculateChange = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'lstItems
'
Me.lstItems.FormattingEnabled = True
Me.lstItems.Items.AddRange(New Object() {"Item 1 - price 5 ", "Item 2 - price 10", "Item 3 - price 7", "Item 4 - price 15"})
Me.lstItems.Location = New System.Drawing.Point(12, 12)
Me.lstItems.Name = "lstItems"
Me.lstItems.Size = New System.Drawing.Size(101, 186)
Me.lstItems.TabIndex = 0
'
'btnCalculate
'
Me.btnCalculate.Location = New System.Drawing.Point(131, 175)
Me.btnCalculate.Name = "btnCalculate"
Me.btnCalculate.Size = New System.Drawing.Size(75, 23)
Me.btnCalculate.TabIndex = 1
Me.btnCalculate.Text = "Calculate"
Me.btnCalculate.UseVisualStyleBackColor = True
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(136, 22)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(73, 13)
Me.Label1.TabIndex = 2
Me.Label1.Text = "Enter number:"
'
'txtNumber
'
Me.txtNumber.Location = New System.Drawing.Point(215, 19)
Me.txtNumber.Name = "txtNumber"
Me.txtNumber.Size = New System.Drawing.Size(100, 20)
Me.txtNumber.TabIndex = 3
'
'Label2
'
Me.Label2.AutoSize = True
Me.Label2.Location = New System.Drawing.Point(155, 48)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(54, 13)
Me.Label2.TabIndex = 2
Me.Label2.Text = "VAT (6%):"
'
'txtVAT
'
Me.txtVAT.Location = New System.Drawing.Point(215, 45)
Me.txtVAT.Name = "txtVAT"
Me.txtVAT.ReadOnly = True
Me.txtVAT.Size = New System.Drawing.Size(100, 20)
Me.txtVAT.TabIndex = 3
'
'Label3
'
Me.Label3.AutoSize = True
Me.Label3.Location = New System.Drawing.Point(128, 74)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(81, 13)
Me.Label3.TabIndex = 2
Me.Label3.Text = "Discount (10%):"
'
'txtDiscount
'
Me.txtDiscount.Location = New System.Drawing.Point(215, 71)
Me.txtDiscount.Name = "txtDiscount"
Me.txtDiscount.ReadOnly = True
Me.txtDiscount.Size = New System.Drawing.Size(100, 20)
Me.txtDiscount.TabIndex = 3
'
'Label4
'
Me.Label4.AutoSize = True
Me.Label4.Location = New System.Drawing.Point(136, 126)
Me.Label4.Name = "Label4"
Me.Label4.Size = New System.Drawing.Size(73, 13)
Me.Label4.TabIndex = 2
Me.Label4.Text = "Enter amount:"
'
'txtAmount
'
Me.txtAmount.Location = New System.Drawing.Point(215, 123)
Me.txtAmount.Name = "txtAmount"
Me.txtAmount.Size = New System.Drawing.Size(100, 20)
Me.txtAmount.TabIndex = 3
'
'Label5
'
Me.Label5.AutoSize = True
Me.Label5.Location = New System.Drawing.Point(175, 100)
Me.Label5.Name = "Label5"
Me.Label5.Size = New System.Drawing.Size(34, 13)
Me.Label5.TabIndex = 2
Me.Label5.Text = "Total:"
'
'txtTotal
'
Me.txtTotal.Location = New System.Drawing.Point(215, 97)
Me.txtTotal.Name = "txtTotal"
Me.txtTotal.ReadOnly = True
Me.txtTotal.Size = New System.Drawing.Size(100, 20)
Me.txtTotal.TabIndex = 3
'
'Label6
'
Me.Label6.AutoSize = True
Me.Label6.Location = New System.Drawing.Point(162, 152)
Me.Label6.Name = "Label6"
Me.Label6.Size = New System.Drawing.Size(47, 13)
Me.Label6.TabIndex = 2
Me.Label6.Text = "Change:"
'
'txtChange
'
Me.txtChange.Location = New System.Drawing.Point(215, 149)
Me.txtChange.Name = "txtChange"
Me.txtChange.ReadOnly = True
Me.txtChange.Size = New System.Drawing.Size(100, 20)
Me.txtChange.TabIndex = 3
'
'btnCalculateChange
'
Me.btnCalculateChange.Enabled = False
Me.btnCalculateChange.Location = New System.Drawing.Point(215, 175)
Me.btnCalculateChange.Name = "btnCalculateChange"
Me.btnCalculateChange.Size = New System.Drawing.Size(100, 23)
Me.btnCalculateChange.TabIndex = 1
Me.btnCalculateChange.Text = "Calculate change"
Me.btnCalculateChange.UseVisualStyleBackColor = True
'
'frmMain
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(342, 225)
Me.Controls.Add(Me.txtChange)
Me.Controls.Add(Me.Label6)
Me.Controls.Add(Me.txtTotal)
Me.Controls.Add(Me.Label5)
Me.Controls.Add(Me.txtDiscount)
Me.Controls.Add(Me.Label3)
Me.Controls.Add(Me.txtVAT)
Me.Controls.Add(Me.Label2)
Me.Controls.Add(Me.txtAmount)
Me.Controls.Add(Me.Label4)
Me.Controls.Add(Me.txtNumber)
Me.Controls.Add(Me.Label1)
Me.Controls.Add(Me.btnCalculateChange)
Me.Controls.Add(Me.btnCalculate)
Me.Controls.Add(Me.lstItems)
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedSingle
Me.MaximizeBox = False
Me.MinimizeBox = False
Me.Name = "frmMain"
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
Me.Text = "Discount Loyal Customers"
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents lstItems As System.Windows.Forms.ListBox
Friend WithEvents btnCalculate As System.Windows.Forms.Button
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents txtNumber As System.Windows.Forms.TextBox
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents txtVAT As System.Windows.Forms.TextBox
Friend WithEvents Label3 As System.Windows.Forms.Label
Friend WithEvents txtDiscount As System.Windows.Forms.TextBox
Friend WithEvents Label4 As System.Windows.Forms.Label
Friend WithEvents txtAmount As System.Windows.Forms.TextBox
Friend WithEvents Label5 As System.Windows.Forms.Label
Friend WithEvents txtTotal As System.Windows.Forms.TextBox
Friend WithEvents Label6 As System.Windows.Forms.Label
Friend WithEvents txtChange As System.Windows.Forms.TextBox
Friend WithEvents btnCalculateChange As System.Windows.Forms.Button
End Class
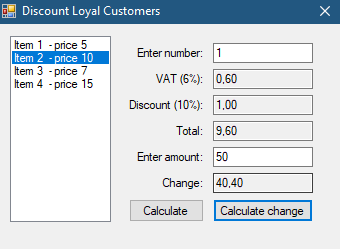
Comments
Leave a comment