package com.company;
import java.util.Scanner;
public class Main {
static Scanner sc = new Scanner(System.in);
//method for finding the sum of two integers
static int sum_of_two_integers(int a, int b)
{
return a + b;
}
//method for finding the division of two integers
static int division_of_two_integers(int a, int b)
{
return a/b;
}
//method for finding the difference of two integers
static int subtraction_of_two_integers(int a, int b)
{
return a - b;
}
//method for finding the product of two integers
static int multiplication_of_two_integers(int a, int b)
{
return a*b;
}
//method called menu to return the option entered
static int menu()
{
System.out.println("Enter your option:");
int option = sc.nextInt();
return option;
}
//The menuControl method
static void menuControl()
{
System.out.println("Enter the operation to perform");
System.out.println("1.Addition");
System.out.println("2.Subtraction");
System.out.println("3.Division");
System.out.println("4.Multiplication");
System.out.println("5.Exit the application");
// call the menu method to enter and return the option
int my_option = menu();
switch(my_option) {
case 1:
System.out.println("Enter the first number");
int first_number = sc.nextInt();
System.out.println("Enter the second number");
int second_number = sc.nextInt();
//call the method for addition
int sum = sum_of_two_integers(first_number,second_number);
System.out.println("The sum of "+first_number+" and "+second_number+" is "+sum);
break;
case 2:
System.out.println("Enter the first number");
int sub_first_number = sc.nextInt();
System.out.println("Enter the second number");
int sub_second_number = sc.nextInt();
//call the method for subtraction
int difference = subtraction_of_two_integers(sub_first_number,sub_second_number);
System.out.println("The sum of "+sub_first_number+" and "+sub_second_number+" is "+difference);
break;
case 3:
System.out.println("Enter the Dividend");
int dividend = sc.nextInt();
System.out.println("Enter the divisor");
int divisor = sc.nextInt();
//call the method for division
int quotient = division_of_two_integers(dividend,divisor);
System.out.println("The Quotient of "+dividend+" and "+divisor+" is "+quotient);
break;
case 4:
System.out.println("Enter the first number");
int multi_first_number = sc.nextInt();
System.out.println("Enter the second number");
int multi_second_number = sc.nextInt();
//call the method for multiplication
int product = multiplication_of_two_integers(multi_first_number,multi_second_number);
System.out.println("The Product of "+multi_first_number+" and "+multi_second_number+" is "+product);
break;
case 5:
System.out.println("Program successfully exited");
break;
default:
System.out.println("Invalid option please try again");
}
}
public static void main(String[] args) {
// call the menuControl method here inside the main method
menuControl();
}
}
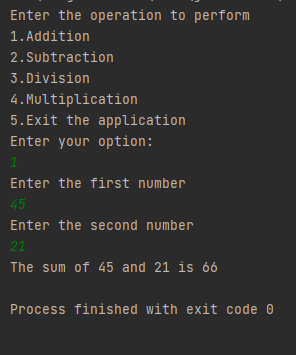
Comments
Leave a comment