import java.util.Scanner;
public class SalesEmployee {
public static void main(String[] args) {
Scanner keyBoard = new Scanner(System.in);
System.out.print("Enter the employee name: ");
String name = keyBoard.nextLine();
float amount = 100;
int saleNumber = 0;
float totalSold = 0;
while (amount != 0) {
amount = -1;
while (amount < 0) {
System.out.print("Enter the sale "+(saleNumber+1)+" amount: ");
amount = keyBoard.nextFloat();
if (amount < 0) {
System.out.println("You have entered a sale less than zero.\nPlease enter the sale again.");
}
}
if(amount!=0) {
totalSold += amount;
saleNumber++;
}
}
float average=totalSold/(float)saleNumber;
System.out.println("---------------------");
System.out.println("EMPLOYEE SALES REPORT");
System.out.println("---------------------");
System.out.println("EMPLOYEE: " + name);
System.out.println("TOTAL SOLD: R " + String.format("%.2f", totalSold));
System.out.println("SALES COUNT: " + saleNumber);
System.out.println("AVERAGE SALES: "+ String.format("%.2f", average));
keyBoard.close();
}
}
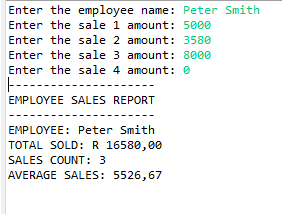
Comments
Leave a comment