#include <iostream>
#include <string>
using namespace std;
//*****************************************
// class Shape declaration *
//*****************************************
class Shape {
public:
void printMyType();
string shapeType;
};
void Shape::printMyType(){
cout<<shapeType;
}
//*****************************************
// class Rectangle declaration *
//*****************************************
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(double w, double h) {
width=w;
height=h;
shapeType = "Rectangle";
}
double calculateArea() {
return width * height;
}
};
//*****************************************
// class Square declaration *
//*****************************************
class Square : public Shape {
private:
double width;
public:
Square(double w) {
width=w;
shapeType = "Square";
}
double calculateArea() {
return width * width;
}
};
int main() {
Rectangle rect1(4, 5);
cout<<"Shape type: ";
rect1.printMyType();
cout<<endl;
cout<<"Area: "<< rect1.calculateArea();
cout<<endl;
return 0;
}
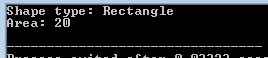
Comments
Leave a comment