#include <iostream>
#include <string>
using namespace std;
class Node
{
public:
int item;
Node* next;
Node(){
this->next = NULL;
}
};
class LinkList
{
public:
Node* head;
//Constructors(default, parameterized, copy) & destructor
LinkList()
{
this->head = NULL;
}
LinkList(Node* head)
{
this->head = head;
}
LinkList(LinkList& linkList)
{
this->head = linkList.head;
}
~LinkList(){
delete head;
}
void PrintList(){
cout<<"Link List Items:\n";
Node* tempNode = this->head;
while (tempNode != NULL)
{
cout<<tempNode->item<<" ";
tempNode = tempNode->next;
}
}
int search_Element(int X){
Node* tempNode = head;
int numberNodes = 0;
while (tempNode != NULL){
if(tempNode->item==X){
return numberNodes;
}
numberNodes++;
tempNode = tempNode->next;
}
return -1;
}
bool Insert_Element(int X){
Node* newNode = new Node();
newNode->item = X;
if (this->head == NULL)
{
this->head = newNode;
}
else
{
Node* tempNode = this->head;
while (tempNode->next != NULL)
{
tempNode = tempNode->next;
}
tempNode->next = newNode;
}
return true;
}
int Length(){
Node* tempNode = head;
int numberNodes = 0;
while (tempNode != NULL)
{
numberNodes++;
tempNode = tempNode->next;
}
return numberNodes;
}
Node* getNodeByPosition(int position)
{
if (position >= 0)
{
Node* currentNode = head;
int i = 0;
for (; i < position; ++i)
{
currentNode = currentNode->next;
}
return currentNode;
}
return NULL;
}
int Insert_Element_at(int X, int pos){
Node* newNode = new Node();
newNode->item = X;
newNode->next = NULL;
if (pos == 0)
{
newNode->next = head;
head = newNode;
}
else if (pos == Length())
{
Insert_Element(X);
}
else
{
Node* prevNode = getNodeByPosition(pos - 1);
Node* nextNode = getNodeByPosition(pos);
prevNode->next = newNode;
newNode->next = nextNode;
return 0;
}
}
int Delete_Element(int X){
if (this->head->item==X)
{
head = head->next;
}
else
{
Node* tempNode = head;
Node* tempPrevious = head;
bool isFound = false;
while (!(isFound = tempNode->item==X) && tempNode->next != NULL)
{
tempPrevious = tempNode;
tempNode = tempNode->next;
}
if (isFound)
{
tempPrevious->next = tempNode->next;
}
else
{
cout<<"Item not found!\n";
}
}
return 0;
}
bool is_Empty(){
return (this->head == NULL);
}
void Print_Reverse_List(){
Node* current = head;
Node *prev = NULL, *next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
head = prev;
PrintList();
}
void Empty_List(){
this->head = NULL;
}
};
int main(){
LinkList* linkList=new LinkList();
int ch=-1;
int number;
int position;
while(ch!=10){
cout<<"1. Insert Element\n";
cout<<"2. Insert Element at position\n";
cout<<"3. Print List\n";
cout<<"4. Search Element\n";
cout<<"5. Delete Element\n";
cout<<"6. Check if List is Empty\n";
cout<<"7. Display Length of List\n";
cout<<"8. Print Reverse List\n";
cout<<"9. Clear List\n";
cout<<"10. Exit\n";
cout<<"Your choice: ";
cin>>ch;
cout<<"\n";
switch(ch){
case 1:
cout<<"Enter the number to be inserted: ";
cin>>number;
linkList->Insert_Element(number);
break;
case 2:
cout<<"Enter the number to be inserted: ";
cin>>number;
cout<<"Enter the position to be inserted: ";
cin>>position;
linkList->Insert_Element_at(number,position);
break;
case 3:
linkList->PrintList();
break;
case 4:
{
cout<<"Enter the number to be searched: ";
cin>>number;
int index=linkList->search_Element(number);
if(index!=-1){
cout<<"The element exists in the position "<<index;
}else{
cout<<"The element DOES NOT exist.";
}
}
break;
case 5:
cout<<"Enter the number to be deleted: ";
cin>>number;
linkList->Delete_Element(number);
cout<<"The number has been deleted";
break;
case 6:
if(linkList->is_Empty()){
cout<<"List is empty";
}else{
cout<<"List is NOT empty";
}
break;
case 7:
cout<<"Length of List is: "<<linkList->Length();
break;
case 8:
linkList->Print_Reverse_List();
break;
case 9:
linkList->Empty_List();
cout<<"List is empty";
break;
case 10:
//exit
delete linkList;
break;
default:
break;
}
cout<<"\n\n";
}
return 0;
}
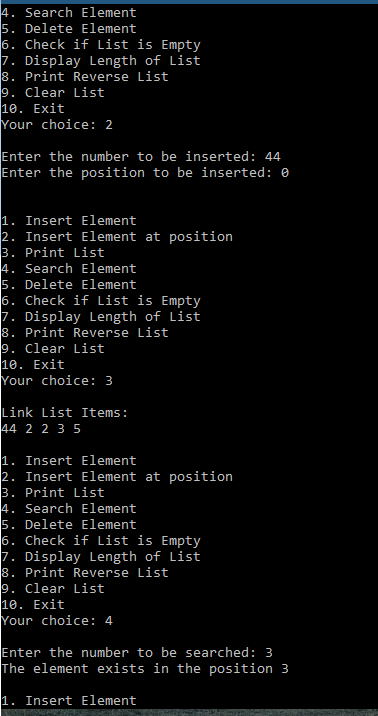
Comments
Leave a comment