#include <iostream>
#include <string>
using namespace std;
#define STUDENTS 3
#define EXAMS 4
void printArrayGrades(const int grades[][EXAMS]);
void findMinimumGrade(const int grades[][EXAMS]);
void findMaximumGrade(const int grades[][EXAMS]);
void findAverageGrade(const int grades[][EXAMS]);
int main(){
int ch = 0;
const int studentGrades[STUDENTS][EXAMS] =
{{ 77, 68, 86, 73 },
{ 96, 87, 89, 78 },
{ 70, 90, 86, 81 }};
while(ch!=4){
cout<<"Enter a choice:\n";
cout<<"0 Print the array of grades\n";
cout<<"1 Find the minimum grade\n";
cout<<"2 Find the maximum grade\n";
cout<<"3 Print the average on all tests for each student\n";
cout<<"4 End program\n";
cout<<"> ";
cin>>ch;
switch(ch){
case 0:
printArrayGrades(studentGrades);
case 1:
findMinimumGrade(studentGrades);
break;
case 2:
findMaximumGrade(studentGrades);
break;
case 3:
findAverageGrade(studentGrades);
break;
case 4:
//exit
break;
default:
break;
}
}
int pause;
cin>>pause;
return 0;
}
void printArrayGrades(const int grades[][EXAMS]){
for(int i = 0; i < STUDENTS; i++){
cout<<"The student "<<(i+1)<<" has the following scores: ";
for(int j = 0; j < EXAMS; j++){
cout<<grades[i][j]<<" ";
}
cout<<"\n";
}
cout<<"\n";
}
void findMinimumGrade(const int grades[][EXAMS]){
int minimumGrade = grades[0][0];
int indexStudent=0;
for(int i = 0; i < STUDENTS; i++){
for(int j = 0; j < EXAMS; j++){
if(grades[i][j]<minimumGrade){
minimumGrade = grades[i][j];
indexStudent=i;
}
}
}
cout<<"The student "<<(indexStudent+1)<<" has the minimum grade: "<<minimumGrade<<"\n";
}
void findMaximumGrade(const int grades[][EXAMS]){
int maximumGrade = grades[0][0];
int indexStudent=0;
for(int i = 0; i < STUDENTS; i++){
for(int j = 0; j < EXAMS; j++){
if(grades[i][j]>maximumGrade){
maximumGrade = grades[i][j];
indexStudent=i;
}
}
}
cout<<"The student "<<(indexStudent+1)<<" has the maximum grade: "<<maximumGrade<<"\n";
}
void findAverageGrade(const int grades[][EXAMS]){
for(int i = 0; i < STUDENTS; i++){
float total = 0;
for(int j = 0; j < EXAMS; j++){
total += grades[ i ][ j ];
}
float average=(float)(total / EXAMS);
cout<<"The average grade for student "<<(i+1)<<" is "<<average<<"\n";
}
}
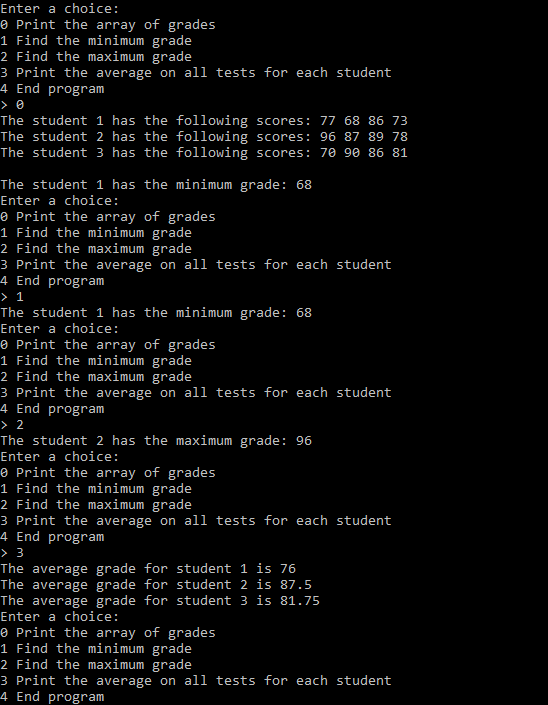
Comments
Leave a comment