#include <iostream>
using namespace std;
/*
Write a Car class that holds the car name , year it was made and speed .The speed should be between 0 (minimum speed) and 225 (maximum speed) .
Include the following functions;
*A function that will display information about the car such as name of the car , year it was made and the current speed.
*A function that will accept an argument,and use that value to increase the speed.if the increase of speed will result to a speed more than the maximum speed ,
the spewed should be kept at the maximum speed.
*A function that will accept an argument , and use that value to decrease the speed .If the decrease of speed will result to a negative speed value ,
the speed should be kept at the minimum speed.
Write a main() function in which you declare one car object , and test (demonstrate) the class works correctly (I.e call the functions.)
*/
#define MIN_SPEED 0
#define MAX_SPEED 225
class Car
{
public:
string Name;
int Model;
int Speed,CurrentSpeed;
void SetData(string s, int m, int cs)
{
Name = s;
Model = m;
CurrentSpeed = cs;
}
void DisplayInfo(void)
{
cout<<"\n\n\tCar Name : "<<Name;
cout<<"\n\tModel : "<<Model;
cout<<"\n\tCurrent Speed : "<<CurrentSpeed;
}
void IncrSpeed(int s)
{
CurrentSpeed = CurrentSpeed+s;
if(CurrentSpeed>MAX_SPEED) CurrentSpeed = MAX_SPEED;
}
void DecrSpeed(int s)
{
CurrentSpeed = CurrentSpeed - s;
if(CurrentSpeed < MIN_SPEED) CurrentSpeed = MIN_SPEED;
}
};
main()
{
class Car C1,C2;
C1.SetData("Alto",2020,85); C1.DisplayInfo();
C2.SetData("Santro",2021,100); C2.DisplayInfo();
C1.IncrSpeed(10); C1.DisplayInfo();
C1.DecrSpeed(20); C1.DisplayInfo();
C2.IncrSpeed(15); C2.DisplayInfo();
C2.DecrSpeed(30); C2.DisplayInfo();
return(0);
}
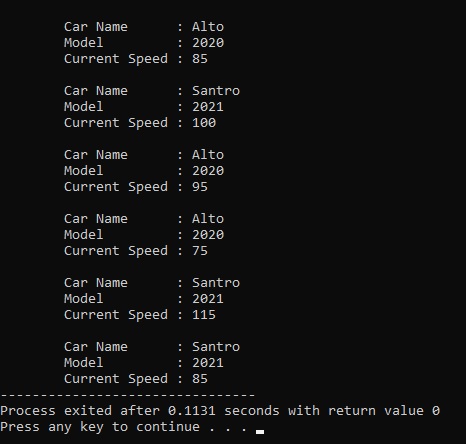
Comments
Leave a comment