using namespace std;
#include <omp.h>
#include <stdio.h>
/*
Create a class named as DeluxPayment that inherit from class Room_Payment and has
following attribute
1. ChargesPerday
This class should have parameterized and default constructor and MakePayment () function which
return the Payment as per given below table
*/
#define NORMAL 0
#define DELUX 1
class RoomPayment
{
public:
int ChargesPerDay_Normal;
int ChargesPerDay_Delux;
void SetCPD(int cpd_n, int cpd_d)
{
ChargesPerDay_Normal = cpd_n;
ChargesPerDay_Delux = cpd_d;
cout<<"\nCharges per day for Normal Room: US $ "<<ChargesPerDay_Normal;
cout<<"\nCharges per day for Delux Room: US $ "<<ChargesPerDay_Delux;
}
};
class DeluxPayment:public RoomPayment
{
public:
int No_of_Days;
int RoomType;
int Payment;
void MakePayment(int n, int rt)
{
No_of_Days=n;
if(rt==NORMAL)
{
Payment = No_of_Days*ChargesPerDay_Normal;
cout<<"\n\nNo. of Days Stay = "<<n;
cout<<"\n\nTotal Payment = "<<n<<" * "<<ChargesPerDay_Normal<<" = "<<n*ChargesPerDay_Normal;
}
if(rt==DELUX)
{
Payment = No_of_Days*ChargesPerDay_Delux;
cout<<"\n\nNo. of Days Stay = "<<n;
cout<<"\nTotal Payment = "<<n<<" * "<<ChargesPerDay_Delux<<" = "<<n*ChargesPerDay_Delux;
}
}
};
int main()
{
class DeluxPayment DP;
DP.SetCPD(200, 300);
DP.MakePayment(2,NORMAL);
DP.MakePayment(5,DELUX);
return(0);
}
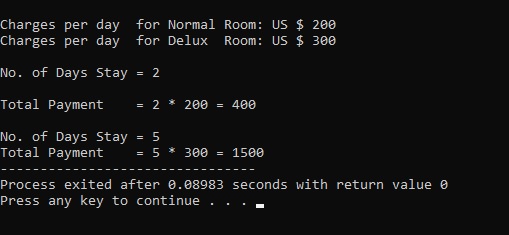
Comments
Leave a comment