The first version of the program uses a switch statement to implement the above program.
#include <iostream>
#include <string>
using namespace std;
int main() {
float amount; // the amount that can be divided between the athletes
char athleteCategory; // the medal recieve: 'G' (gold) or 'S' (silver) or 'B' (bronze) or 'P'(places)
float cashBonus;
cout << "Enter amount: ";
cin >> amount;
cout << "Enter Athlete Category: ";
cin>>athleteCategory;
switch(athleteCategory){
case 'G':
//A gold medalist gets a 15% cash bonus.
cashBonus=amount*0.15;
break;
case 'S':
//A silver medalist gets a 12% cash bonus and
cashBonus=amount*0.12;
break;
case 'B':
//A bronze medalist gets a 10% cash bonus.
cashBonus=amount*0.1;
break;
case 'P':
//All the other athletes get a 5% cash bonus if they are placed among the first 6 athletes.
cashBonus=amount*0.05;
break;
default:
break;
}
amount+=cashBonus;
//displays the final amount that is due, after discount.
cout<<"The cash bonus: "<<cashBonus<<"\n";
cout<<"The final amount that is due: "<<amount<<"\n";
system("pause");
return 0;
}
The second version of the program uses nested-if statements.
#include <iostream>
#include <string>
using namespace std;
int main() {
float amount; // the amount that can be divided between the athletes
char athleteCategory; // the medal recieve: 'G' (gold) or 'S' (silver) or 'B' (bronze) or 'P'(places)
float cashBonus;
cout << "Enter amount: ";
cin >> amount;
cout << "Enter Athlete Category: ";
cin>>athleteCategory;
if(amount>0){
if(athleteCategory== 'G'){
//A gold medalist gets a 15% cash bonus.
cashBonus=amount*0.15;
}
if(athleteCategory== 'S'){
//A silver medalist gets a 12% cash bonus and
cashBonus=amount*0.12;
}
if(athleteCategory== 'B'){
//A bronze medalist gets a 10% cash bonus.
cashBonus=amount*0.1;
}
if(athleteCategory== 'P'){
//All the other athletes get a 5% cash bonus if they are placed among the first 6 athletes.
cashBonus=amount*0.05;
}
}
amount+=cashBonus;
//displays the final amount that is due, after discount.
cout<<"The cash bonus: "<<cashBonus<<"\n";
cout<<"The final amount that is due: "<<amount<<"\n";
system("pause");
return 0;
}
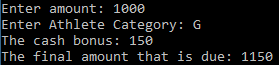
Comments
Leave a comment