#include<iostream>
#include<bits/stdc++.h>
using namespace std;
class inputData{
int data_a,data_b;
public:
void input()
{
cout<<"Enter value of a : ";
cin>>data_a;
cout<<"Enter value of b : ";
cin>>data_b;
}
void display()
{
cout<<"Value of a : ";
cout<<data_a;
cout<<"Value of b : ";
cout<<data_b;
}
int get_a()
{
return data_a;
}
int get_b()
{
return data_b;
}
};
class Arith_Unit:public inputData{
public:
int add()
{
int n = get_a();
int m = get_b();
return n + m;
}
int sub()
{
int n = get_a();
int m = get_b();
return n - m;
}
int mul()
{
int n = get_a();
int m = get_b();
return n * m;
}
int div()
{
int n = get_a();
int m = get_b();
return n / m;
}
};
class Logic_Unit: public inputData{
public:
int And()
{
int n = get_a();
int m = get_b();
return n&m;
}
int Or()
{
int n = get_a();
int m = get_b();
return n|m;
}
int Xor()
{
int n = get_a();
int m = get_b();
return n^m;
}
};
int main()
{
Arith_Unit ob1;
ob1.input();
cout<<"1. Add"<<endl<<"2. Subtract"<<endl<<"3. Multiply"<<endl<<"4. Divide"<<endl<<endl;
cout<<"Enter which operation to perform : ";
int option;
cin>>option;
switch(option)
{
case 1:
cout<<ob1.get_a()<<" + "<<ob1.get_b()<<" = "<<ob1.add()<<endl;
break;
case 2:
cout<<ob1.get_a()<<" - "<<ob1.get_b()<<" = "<<ob1.sub()<<endl;
break;
case 3:
cout<<ob1.get_a()<<" * "<<ob1.get_b()<<" = "<<ob1.mul()<<endl;
break;
case 4:
cout<<ob1.get_a()<<" / "<<ob1.get_b()<<" = "<<ob1.div()<<endl;
break;
}
cout<<endl;
Logic_Unit ob2;
ob2.input();
cout<<"1. And"<<endl<<"2. Or"<<endl<<"3. Xor"<<endl<<endl;
cout<<"Enter which operation to perform : ";
int option1;
cin>>option1;
switch(option1)
{
case 1:
cout<<ob1.get_a()<<" & "<<ob1.get_b()<<" = "<<ob2.And()<<endl;
break;
case 2:
cout<<ob1.get_a()<<" | "<<ob1.get_b()<<" = "<<ob2.Or()<<endl;
break;
case 3:
cout<<ob1.get_a()<<" ^ "<<ob1.get_b()<<" = "<<ob2.Xor()<<endl;
break;
}
}
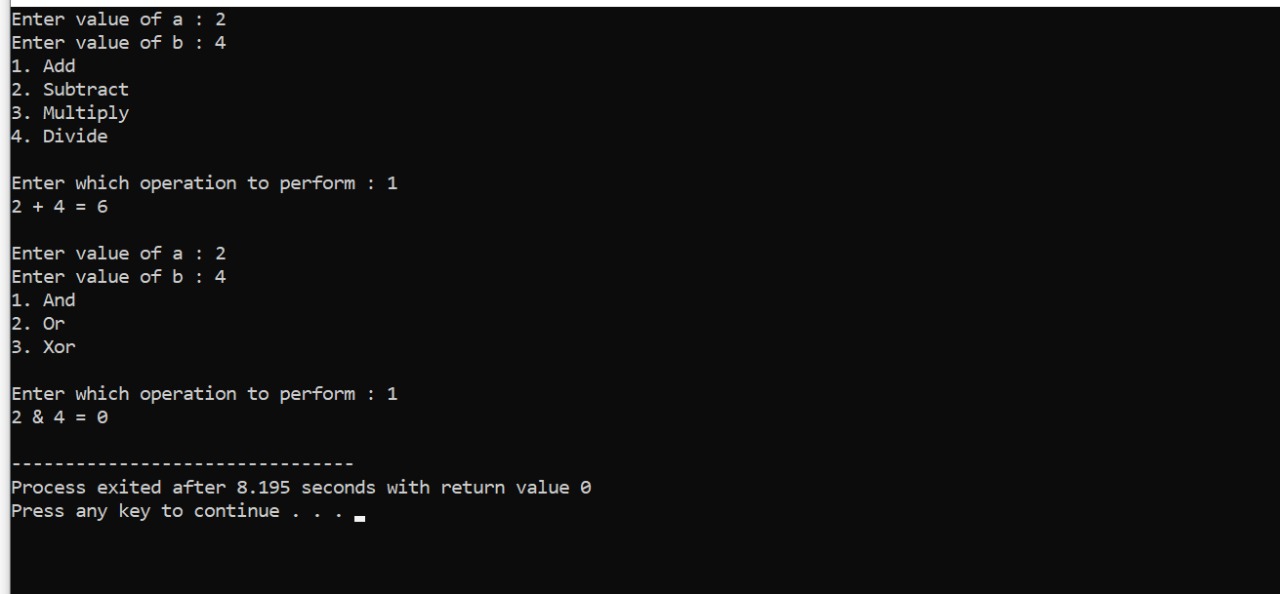
Comments
Leave a comment