#include <iostream>
using namespace std;
class FloatArray;
//Create two classes IntArray to store the set of integer numbers and FloatArray to store decimal numbers.
class IntArray{
private:
int numbers[5];
public:
//Add a member function read() for both classes for reading inputs.
void read(){
for(int i=0;i<5;i++){
cout<<"Enter integer value "<<(i+1)<<": ";
cin>>numbers[i];
}
}
friend void maxmin(IntArray x,FloatArray y);
};
class FloatArray{
private:
float numbers[5];
public:
//Add a member function read() for both classes for reading inputs.
void read(){
for(int i=0;i<5;i++){
cout<<"Enter decimal value "<<(i+1)<<": ";
cin>>numbers[i];
}
}
//Using a friend function maxmin(x,y), display the maximum and
//minimum among the set of integers and decimal numbers.
friend void maxmin(IntArray x,FloatArray y);
};
void maxmin(IntArray x,FloatArray y){
float min=x.numbers[0];
float max=x.numbers[0];
for(int i=0;i<5;i++){
if(x.numbers[i]<min){
min=x.numbers[i];
}
if(y.numbers[i]<min){
min=y.numbers[i];
}
if(x.numbers[i]>max){
max=x.numbers[i];
}
if(y.numbers[i]>max){
max=y.numbers[i];
}
}
cout<<"\nThe minimum value is: "<<min<<"\n";
cout<<"\nThe maximum value is: "<<max<<"\n";
}
int main () {
//Create two objects ‘x’ for IntArray and ‘y’ for FloatArray.
//Read the inputs for x and y.
IntArray x;
FloatArray y;
x.read();
cout<<"\n";
y.read();
maxmin(x,y);
//Delay
system("pause");
return 0;
}
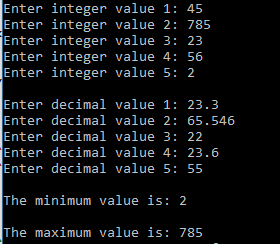
Comments
Leave a comment