#include <iostream>
#include <string>
#include <vector>
using namespace std;
//Create a structure to specify data of customers in a bank. The data to be stored is:
struct Customer{
int accountNumber;//Account number
string name;//Name
float balanceAccount;//Balance in account
};
//a function to print the Account number and name of each customer with balance below Rs. 100.
void printCustomersBalanceBelow100(struct Customer customers[200],int totalCustomers){
cout<<"\n\nCustomer Details whose Balance < 100 Rs.\n";
for(int i=0;i<totalCustomers;i++){
if(customers[i].balanceAccount<100)
{
cout<<"Customer account number: "<<customers[i].accountNumber<<"\n";
cout<<"Customer name: "<<customers[i].name<<"\n";
cout<<"Customer balance: "<<customers[i].balanceAccount<<"\n\n";
}
}
cout<<"\n";
}
int main(){
//Assume maximum of 200 customers in the bank.
struct Customer customers[200];
customers[0].accountNumber=1;
customers[0].name="Mary Clark";
customers[0].balanceAccount=0;
customers[1].accountNumber=2;
customers[1].name="Mike Smith";
customers[1].balanceAccount=0;
customers[2].accountNumber=3;
customers[2].name="Peter Clark";
customers[2].balanceAccount=0;
customers[3].accountNumber=4;
customers[3].name="John Willams";
customers[3].balanceAccount=0;
int totalCustomers=4;
int code;
for(int i=0;i<totalCustomers;i++){
int accountNumber;
cout<<"Enter the customer account number: ";
cin>>accountNumber;
int index=-1;
for (int c = 0; c < totalCustomers; c++){
if (customers[c].accountNumber == accountNumber){
index=i;
break;
}
}
if(index!=-1){
code=-1;
while(code<0 || code>1){
cout<<"Enter code 1 - deposit, 0 - withdrawal: ";
cin>>code;
}
float amount;
if (code)
{
cout<<"Enter amount to be deposit: ";
cin>>amount;
customers[i].balanceAccount += amount;
cout<<"\nAccount balance is: "<< customers[i].balanceAccount<<"\n\n";
}
else
{
printf("Enter amount to withdraw: ");
cin>>amount;
if (customers[i].balanceAccount - amount < 100){
cout<<"\nThe balance is insufficient for the specified withdrawal.\n\n";
}else{
customers[i].balanceAccount -= amount;
cout<<"\nThe account balance is: "<< customers[i].balanceAccount<<"\n\n";
}
}
}else{
cout<<"\nThe account number does not exist.\n\n";
}
}
printCustomersBalanceBelow100(customers,totalCustomers);
system("pause");
return 0;
}
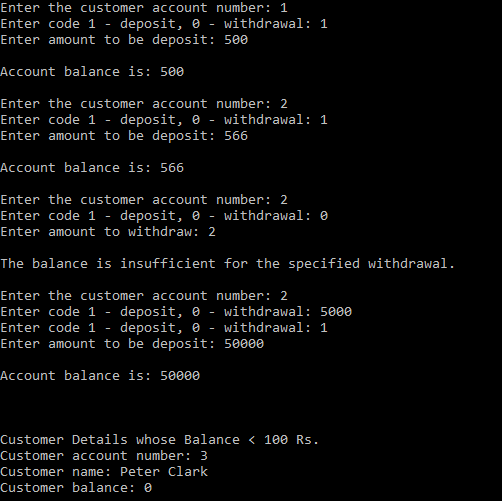
Comments
Leave a comment