#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int frequency (int arr[], int size) {
bool check[size];
for(int i = 0; i < size; i++){
check[i] = 0;
}
for(int i = 0; i < size; i++){
if(check[i] == 1){
continue;
}
int count = 1;
for(int j = i + 1; j < size; j++){
if (arr[i] == arr[j]){
check[j] = 1;
count++;
}
}
cout<<"frequency of "<<arr[i]<<" is: " << count << endl;
}
}
int main () {
srand(time(NULL));
int number_students = 300;
int minumum_mark = 60;
// we declare an array for store marks
int marks[number_students];
// we fill the array randomly 0 to 100 marks
for (int i = 0; i < number_students; i++) {
marks[i] = rand() % 101;
}
// we store marks that greater than 60 to array
cout << "marks greater than 60: " << endl;
int n = 0;
int marks_60[n];
for (int i = 0; i < number_students; i++) {
if (marks[i] >= 60) {
marks_60[n++] = marks[i];
cout << marks[i] << " ";
}
}
// we sort the marks_60[n] array
cout << "\nthe sorted marks greater than 60: ";
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (marks_60[i] > marks_60[j]) {
int temp;
temp = marks_60[i];
marks_60[i] = marks_60[j];
marks_60[j] = temp;
}
}
}
for (int i = 0; i < n; i++) {
cout << marks_60[i] << " ";
}
cout << endl;
frequency(marks_60, n);
return 0;
}
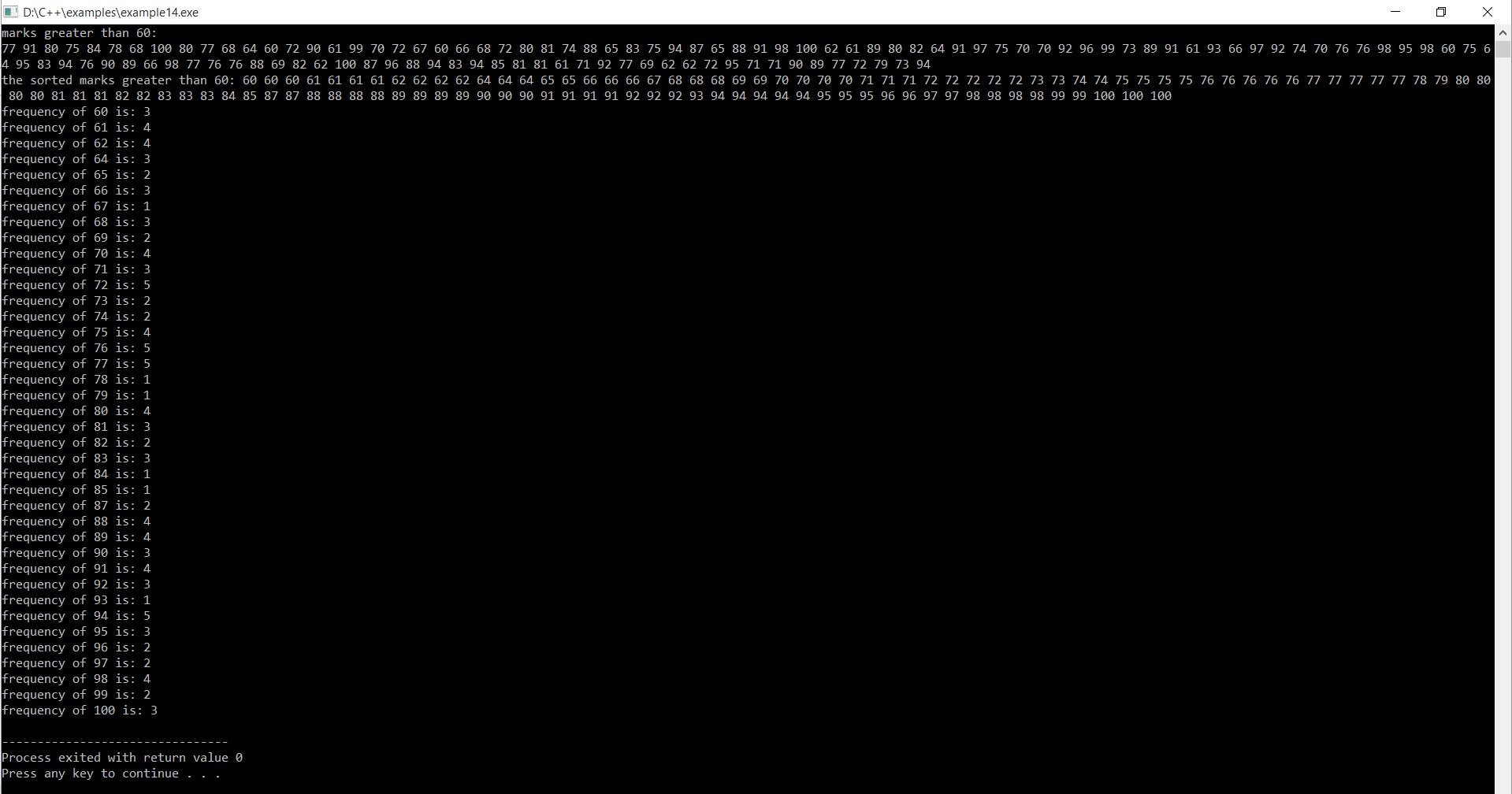
Comments
Leave a comment