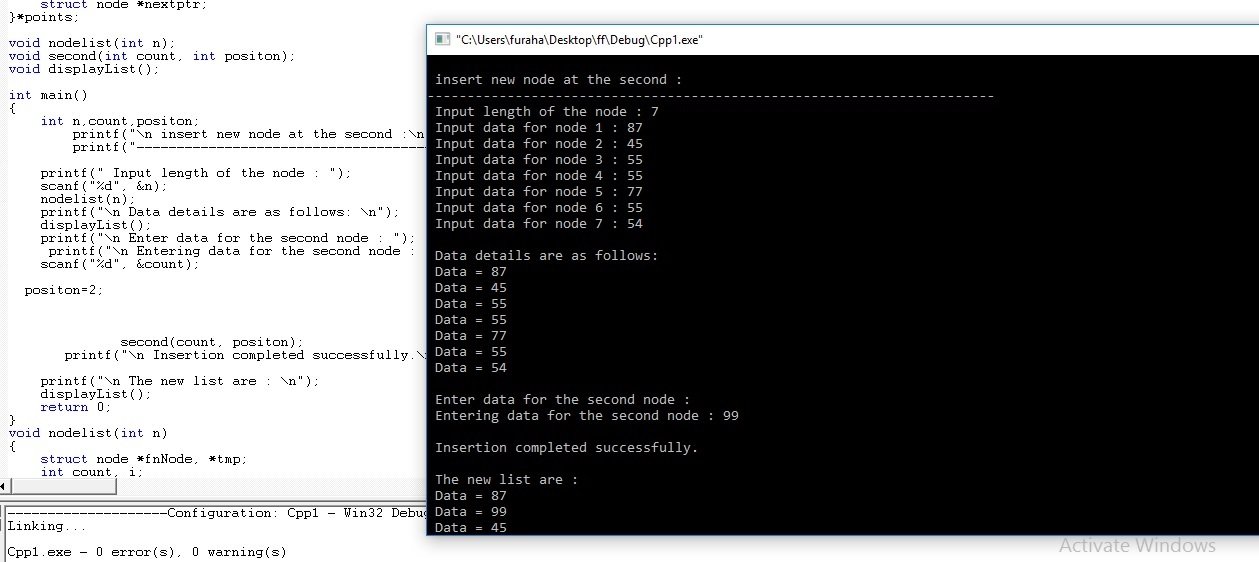
#include <stdio.h>
#include <stdlib.h>
struct node
{
int count;
struct node *nextptr;
}*points;
void nodelist(int n);
void second(int count, int positon);
void displayList();
int main()
{
int n,count,positon;
printf("\n insert new node at the second :\n");
printf("-----------------------------------------------------------------------\n");
printf(" Input length of the node : ");
scanf("%d", &n);
nodelist(n);
printf("\n Data details are as follows: \n");
displayList();
printf("\n Enter data for the second node : ");
printf("\n Entering data for the second node : ");
scanf("%d", &count);
positon=2;
second(count, positon);
printf("\n Insertion completed successfully.\n ");
printf("\n The new list are : \n");
displayList();
return 0;
}
void nodelist(int n)
{
struct node *fnNode, *tmp;
int count, i;
points = (struct node *)malloc(sizeof(struct node));
if(points == NULL)
printf(" Memory can not be allocated.");
}
else
{
printf(" Input data for node 1 : ");
scanf("%d", &count);
points-> count = count;
points-> nextptr = NULL;
tmp = points;
for(i=2; i<=n; i++)
{
fnNode = (struct node *)malloc(sizeof(struct node));
if(fnNode == NULL)
{
printf(" Memory can not be allocated.");
break;
}
else
{
printf(" Input data for node %d : ", i);
scanf(" %d", &count);
fnNode->count = count; // links the count field of fnNode with count
fnNode->nextptr = NULL; // links the address field of fnNode with NULL
tmp->nextptr = fnNode; // links previous node i.e. tmp to the fnNode
tmp = tmp->nextptr;
}
}
}
}
void second(int count, int positon)
{
int i;
struct node *fnNode, *tmp;
fnNode = (struct node*)malloc(sizeof(struct node));
if(fnNode == NULL)
{
printf(" Memory can not be allocated.");
}
else
{
fnNode->count = count;
fnNode->nextptr = NULL;
tmp = points;
for(i=2; i<=positon-1; i++)
{
tmp = tmp->nextptr;
if(tmp == NULL)
break;
}
if(tmp != NULL)
{
fnNode->nextptr = tmp->nextptr;
tmp->nextptr = fnNode;
}
else
{
printf(" Insert is not positonsible to the given positonition.\n");
}
}
}
void displayList()
{
struct node *tmp;
if(points == NULL)
{
printf(" No data found in the empty list.");
}
else
{
tmp = points;
while(tmp != NULL)
{
printf(" Data = %d\n", tmp->count);
tmp = tmp->nextptr;
}
}
}
Comments
Leave a comment